11. Design principles and patterns
Table of Contents
A. SOLID principles
A mnemonic for 5 design principles of object-oriented programs.
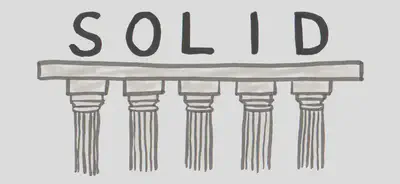
-
Single responsibility principle (SRP): A class, module, or function in a program should do only one job.
- If a single functionality breaks, you know where the bug will be in the code and can trust that only that class will break.
-
Open-closed principle (OCP): A program should be open for extension, but closed for modification.
- create entities that can be widely adapted but also remain unchanged.
- creating duplicate entities with specialized behavior through polymorphism.
- create entities that can be widely adapted but also remain unchanged.
-
Liskov substitution principle (LSP): Objects in a program should be replaceable with instances of their subtypes without altering the correctness of that program.
- In simpler words, any class must be directly replaceable by any of its subclasses without error.
-
Interface segregation principle (ISP): Many client-specific interfaces are better than one general-purpose interface.
Any unused part of the method should be removed or split into a separate method.
-
Dependency inversion principle (DIP): One should depend upon abstractions, [not] concretions.
- High level modules should not depend upon low level modules. Both should depend upon abstractions.
- Abstractions should not depend on details. Details should depend on abstractions.
If you minimize dependencies, changes will be more localized and require less work to find all affected components.
- DIP decouples high and low-level components and instead connects both to abstractions.
Summary
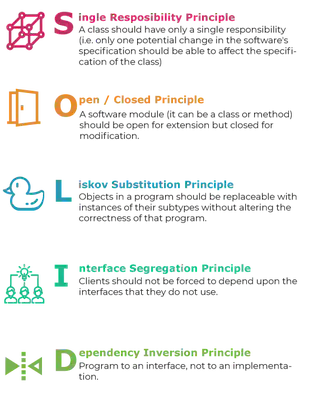
A1. Achieve Low Coupling
-
Avoid deep inheritance
- Seperate creating resources from using them
-
Introduce abstractions
- Use interfaces and abstract classes
-
Avoid inappropriately intimacy
- What is it? Getting a lot more data than the required data.
-
Introduce an intermediate data structure
B. Technical Debt
Often technical debt refers to a rushed development process or a lack of shared knowledge among team members. Sometimes it is inevitable.
- Knowledge based debt
- Design based debt
- Code based debt
B1. How to tackle technical debt
- Code and architecture refactoring - Resolve code and design debt is organising a refactoring week every X sprints
- Start regular technical debt discussions
- Start tracking technical debt in your editor
- Documentation
- Code comments
- JIRA tickets (backlogs)